5 Ways to Append Items to an Array
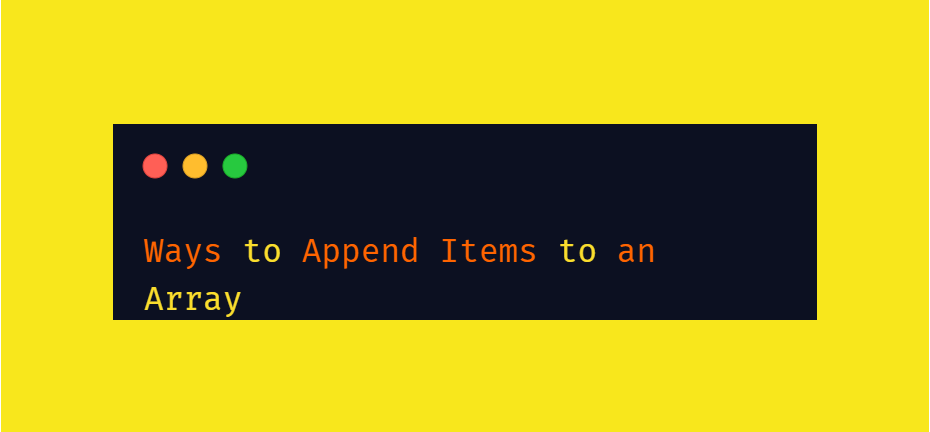
Arrays are the workhorses of programming. They allow us to store and manipulate collections of data, making them an essential part of almost every software application. One common operation we often need to perform on arrays is appending items. But what are the best ways to accomplish this task? In this blog post, we will explore five different methods to append items to an array. We'll provide examples and discuss when each method is most suitable. So, let's dive in and enhance our array-manipulation skills!
1. Using the push()
Method
One of the simplest and most common ways to append an item to an array is by using the push()
method. This method adds one or more elements to the end of an array and returns the new length of the array. It's like adding new passengers to the back of a bus.
const fruits = ["apple", "banana", "cherry"];
fruits.push("date");
console.log(fruits); // Output: ["apple", "banana", "cherry", "date"]
This method is effective when you want to add elements to the end of an array and don't need to specify a particular index. It's efficient and easy to use, just like hopping on the last carriage of a train.
Unveiling the Magic of -> CSS :not Selector
2. Using the Spread Operator ([...array, newItem]
)
The spread operator is another fantastic way to append an item to an array. It creates a new array that includes the elements of the existing array, followed by the new item. Think of it as creating a new deck of cards by shuffling in a new one.
const cities = ["New York", "Los Angeles", "Chicago"];
const newCity = "Miami";
const updatedCities = [...cities, newCity];
console.log(updatedCities); // Output: ["New York", "Los Angeles", "Chicago", "Miami"]
This approach is great for when you want to keep the original array intact and create a modified copy with the new element added. It's like adding a twist to your favorite recipe while preserving the original.
Ways to Center Elements with Flexbox
3. Using concat()
Method
If you prefer a more traditional method for appending items, you can use the concat()
method. This method merges two or more arrays and returns a new array, without modifying the original arrays. It's similar to creating a mixtape by combining your favorite songs from different albums.
const array1 = [1, 2, 3];
const array2 = [4, 5];
const newArray = array1.concat(array2);
console.log(newArray); // Output: [1, 2, 3, 4, 5]
This technique is suitable when you want to append items from another array without changing the original arrays. It's like borrowing a book from the library without altering the book itself.
String - charAt() Method in JavaScript
4. Using the Index
Appending items to an array by directly accessing a specific index is another approach. This method is useful when you want to insert an element at a particular position within the array. It's like choosing your seat on an airplane.
const colors = ["red", "blue", "green"];
colors[1] = "yellow"; // Replace the item at index 1
console.log(colors); // Output: ["red", "yellow", "green"]
By assigning a new value to an existing index, you can effectively append or replace items in the array. This method offers precise control over where your new item lands, just like choosing the perfect spot for your tent while camping.
Ways to Convert String to Character Array in JavaScript
5. Using the unshift()
Method
The unshift()
method is the counterpart of push()
. Instead of adding elements to the end of an array, it adds them to the beginning. It's like welcoming passengers onto a bus from the front entrance.
const animals = ["lion", "tiger"];
animals.unshift("elephant");
console.log(animals); // Output: ["elephant", "lion", "tiger"]
This method is useful when you need to prepend an item to an array, such as when maintaining a history of actions. It allows you to place new elements at the start of your array, just like being at the front of the line for a concert.
Ways to Merge Arrays in JavaScript 🎉
Conclusion
In the world of programming, arrays are our trusty companions, and knowing how to append items to them is a fundamental skill. We've explored five different methods to achieve this: push()
, the spread operator, concat()
, using the index, and unshift()
. Each method has its own strengths and use cases, like different tools in a toolbox.
Pretty JSON Output: Making Your Data Shine
So, which method is the best? Well, it depends on the specific requirements of your project. If you want to add items to the end of an array, push()
or the spread operator will serve you well. If you need a modified copy of the array with the new item, the spread operator or concat()
is your friend. If you require precise control over the position of the new item, directly accessing the index is the way to go. And if you want to add items to the beginning of the array, unshift()
is your choice.
How to Set Dynamic Property Keys with ES6 ?
Remember, just as a skilled carpenter selects the right tool for the job, a skilled programmer chooses the best method for their specific task. So, go ahead and append items to arrays with confidence, knowing you have multiple techniques at your disposal. Happy coding!
Events Handling In JavaScript🎉 ,
How to Check if Object is Empty in JavaScript